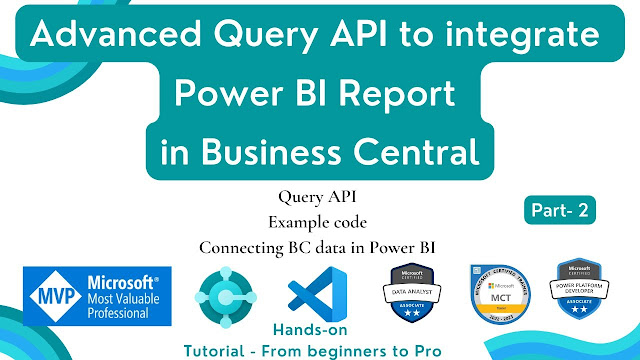
Introduction
The most effective procedures for creating AL code for Microsoft Dynamics 365 Business Central. It covers several topics, including:
- Naming conventions: The recommendations advise using camel case for local variable names and Pascal case for variable and function names. They also recommend using a specific naming convention for functions, such as "FunctionName(InputType1: Type1, InputType2: Type2): ReturnType".
- Formatting: The guidelines recommend using a consistent indentation and spacing style throughout your code, and placing the opening curly brace of a function on the same line as the function name.
- Comments: The guidelines recommend providing clear and concise comments throughout your code, explaining the purpose of each function, variable, and other elements.
- Error handling: The guidelines recommend using the "ERROR" function to handle errors and exceptions, rather than using the "raise" statement.
- Performance: The guidelines recommend being mindful of performance when designing and implementing your code. They recommend using efficient algorithms and avoiding unnecessary calculations, data retrieval, and data manipulation.
- Security: The guidelines recommend following best practices for data validation, user input validation, and error handling to ensure that your code is secure.
- Testability: The guidelines recommend designing your code in such a way that it is easy to test and debug. This includes keeping functions small and focused, with a single purpose and minimal dependencies.
- Reusability: The guidelines recommend designing your code in such a way that it can be easily reused in other parts of the system, or in other systems.
- Compatibility: The guidelines recommend testing your code in different environments, and with different versions of the Dynamics 365 Business Central platform, to ensure compatibility.
- Compliance: The guidelines recommend adhering to local laws, regulations, and industry standards when developing your code.
These guidelines are a great set of general direction to
follow when developing code in AL, following them will help ensure your code is
maintainable, readable, and easy to understand, and also that it performs well
and comply with industry standards.
Examples
Here are some examples that demonstrate the best practices for AL code development outlined in the guidelines from the website you provided:
Naming conventions:
// Pascal casing for variable and function names
MyGlobalVariable: Integer;
CalculateTotal() : Decimal;
// camel casing for local variable names
localMyVariable : Boolean;
Formatting
Comments
// Clear and concise comments
// The cost of a list of things is calculated overall by this function.
function CalculateTotal(items : List of Item) : Decimal
begin
// some code here
end;
Error handling
// Using the ERROR function to handle errors
if (Variable1 > Variable2) then
begin
ERROR('Variable1 must be less than or equal to Variable2');
end;
Performance
Security
Testability
Reusability
Compatibility
Compliance
Here are some additional points for each of the best practices for AL code development outlined in the guidelines:
- Naming conventions:
- Always choose meaningful and descriptive names for variables, functions, and other elements.
- Abbreviations should be avoided unless they are commonly used and well-understood by others.
- Avoid using single letters as variable names, except for indexing loops.
- Formatting:
- Use consistent indentation throughout your code, to indicate the hierarchy of functions and other elements.
- Use consistent spacing between elements, such as operators, commas, and curly braces.
- Use a consistent style for punctuation and capitalization, such as always including semicolons at the end of statements.
- Comments:
- Comments should provide a high-level explanation of the purpose of the code and its key elements.
- Avoid using comments to simply repeat what the code is doing.
- Commenting on "how" code works is less important than why it works.
- Use the appropriate commenting style for the language you're using, such as "//" for AL
- Error handling:
- Use the ERROR function to handle errors, rather than using the "raise" statement. This provides a consistent way of handling errors throughout your code.
- Define a clear and consistent error-handling strategy for your code.
- Always provide a clear error message that describes the problem and how to fix it.
- Performance:
- Avoid unnecessary calculations and data retrieval.
- Be mindful of the performance implications of algorithms and data structures.
- Identify and optimize performance bottlenecks in your code using profiling tools.
- Security:
- Validate all input data to ensure that it is of the expected type, format, and value.
- Sanitize input data to prevent SQL injection, cross-site scripting (XSS), and other types of attacks.
- Use secure cryptographic techniques to protect sensitive data, such as passwords and other authentication information.
- Error messages should not disclose sensitive information
- Testability:
- Design your code to be easy to test and debug.
- Keep functions small and focused, with a single purpose and minimal dependencies.
- Use a consistent testing strategy throughout your code.
- Use automated testing frameworks to test your code.
- Reusability:
- Design your code to be easily reusable in other parts of the system or other systems.
- Use modular design principles, such as encapsulation and abstraction.
- Keep functions and other elements as generic as possible, so that they can be reused in different contexts.
- Provide clear and detailed documentation for reusable functions and other elements
- Compatibility:
- Test your code in different environments and with different versions of the Dynamics 365 Business Central platform, to ensure compatibility.
- Test your code with different configurations of the platform, such as different locales and currencies.
- Keep track of changes to the platform and make sure your code is compatible with the latest version.
- Compliance:
- Adhere to local laws, regulations, and industry standards when developing your code.
- Use industry-standard libraries and frameworks whenever possible.
- Keep track of changes in compliance regulations and ensure that your code is up to date.
These are just a few of the many considerations that should
be taken into account when developing code in AL and Microsoft Dynamics 365
Business Central. Remember to always strive for readability, maintainability,
and good performance when creating your code to allow future developers to
quickly understand and maintain it.
0 Comments